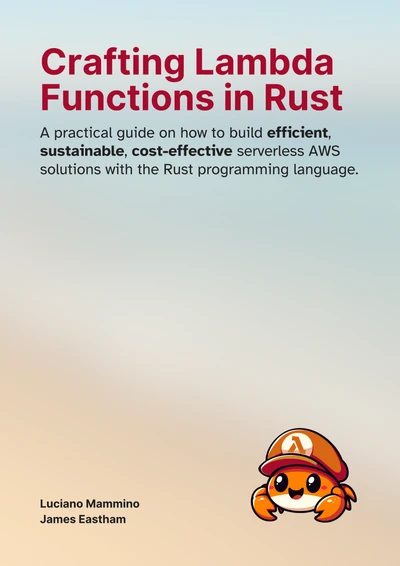
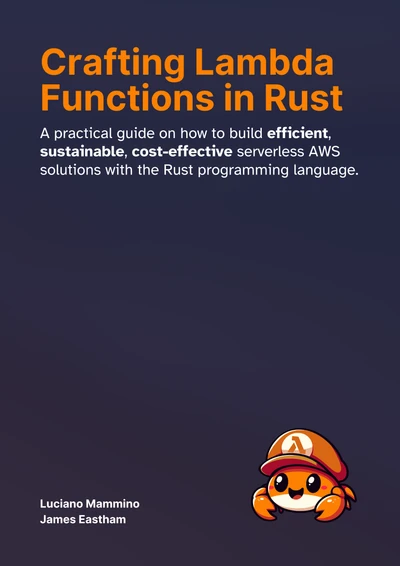
Crafting Lambda Functions in Rust
A practical guide on how to build efficient, sustainable, cost-effective serverless AWS solutions with the Rust programming language.
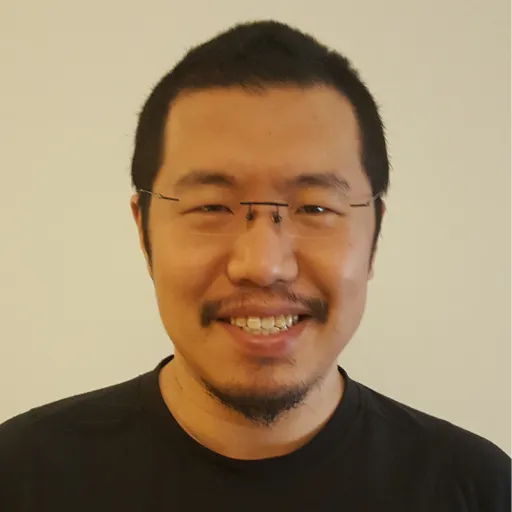
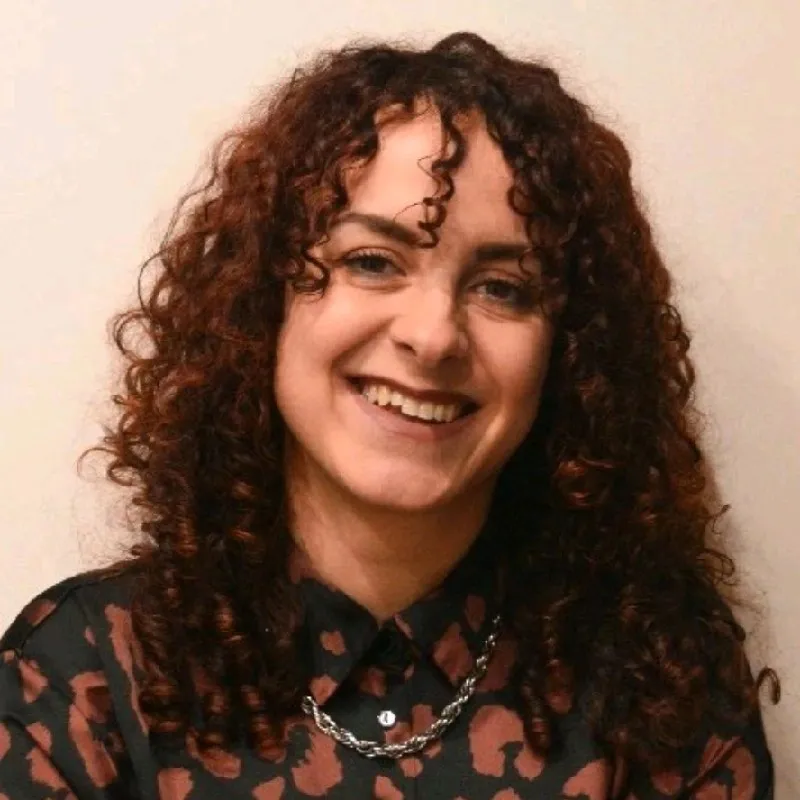
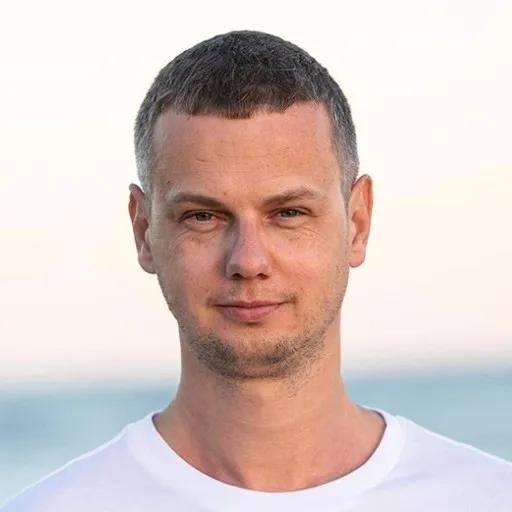
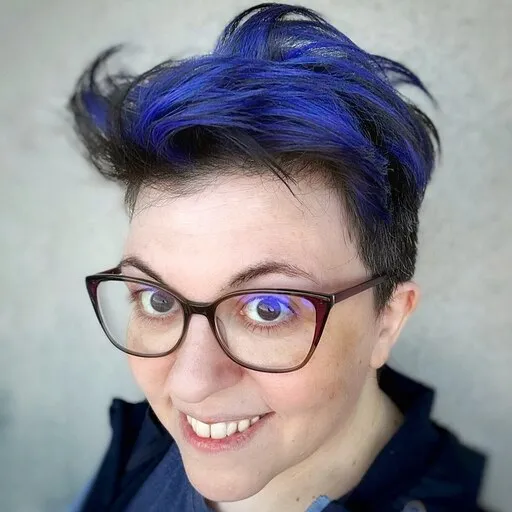
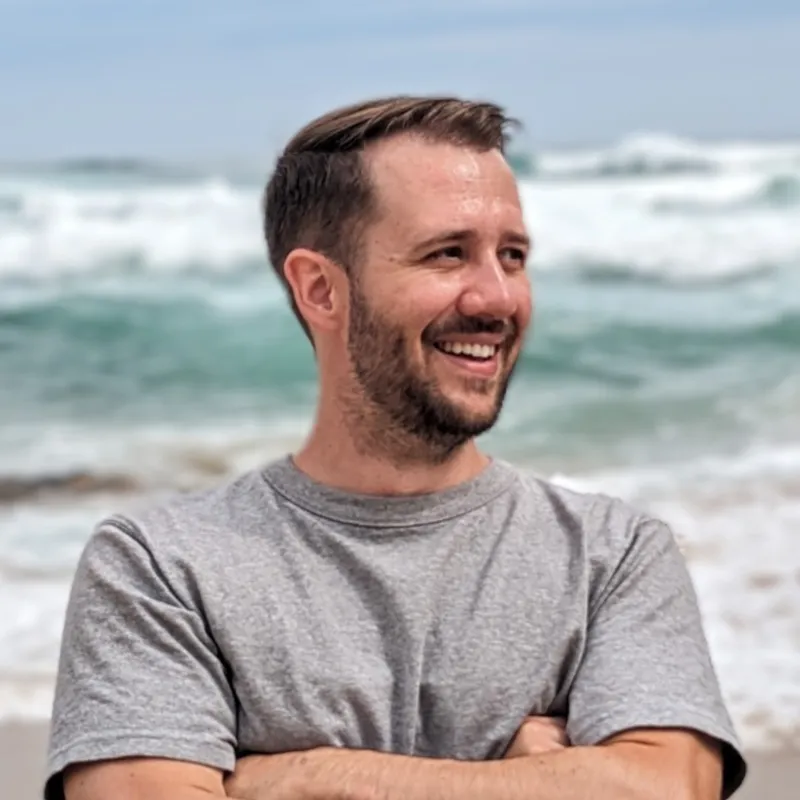
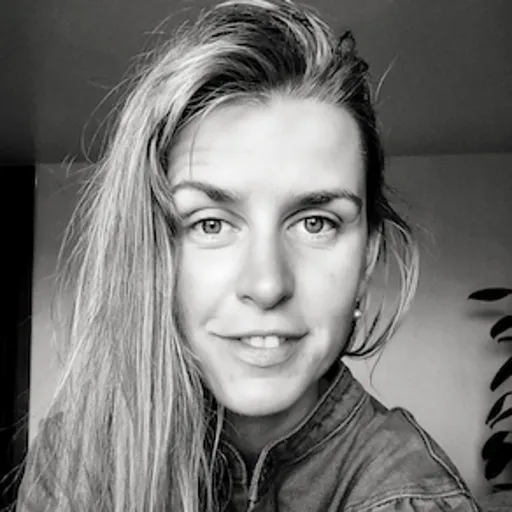
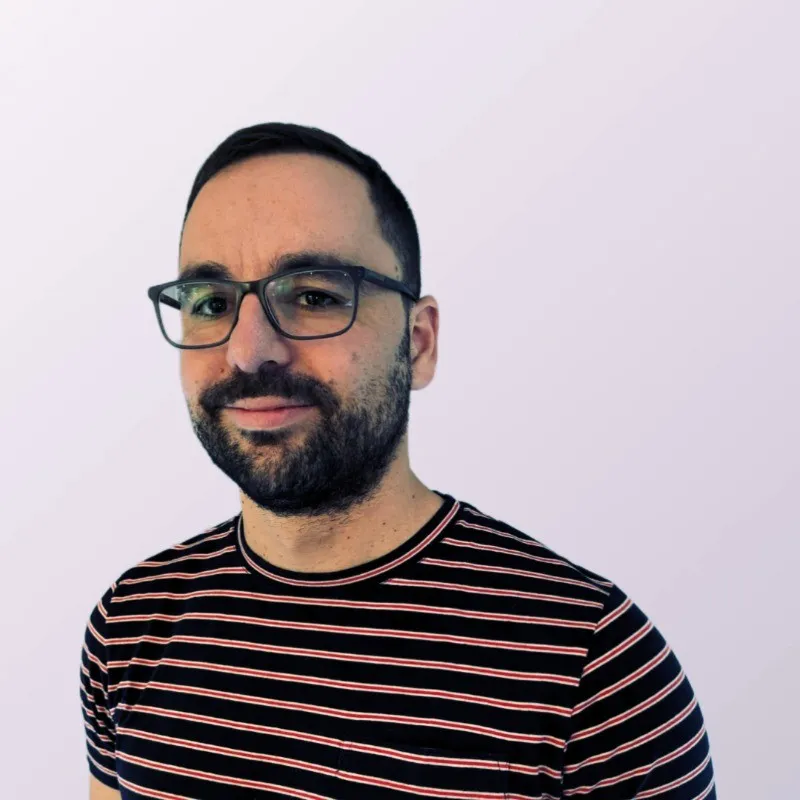
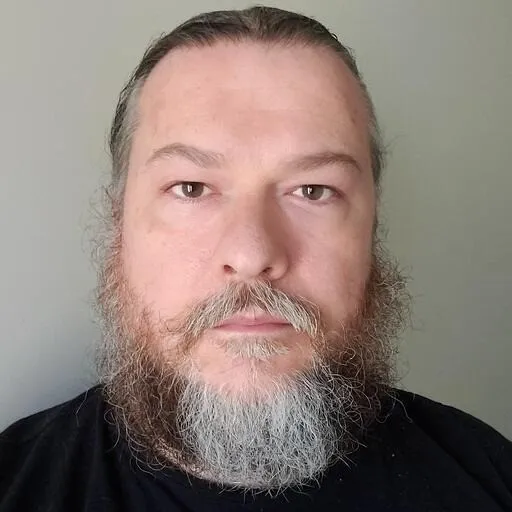
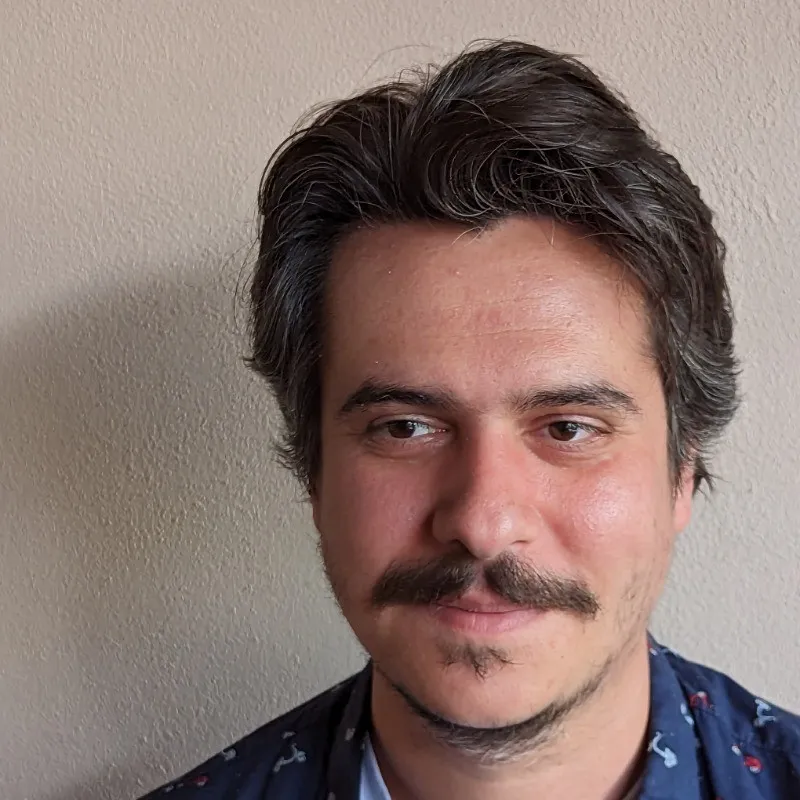
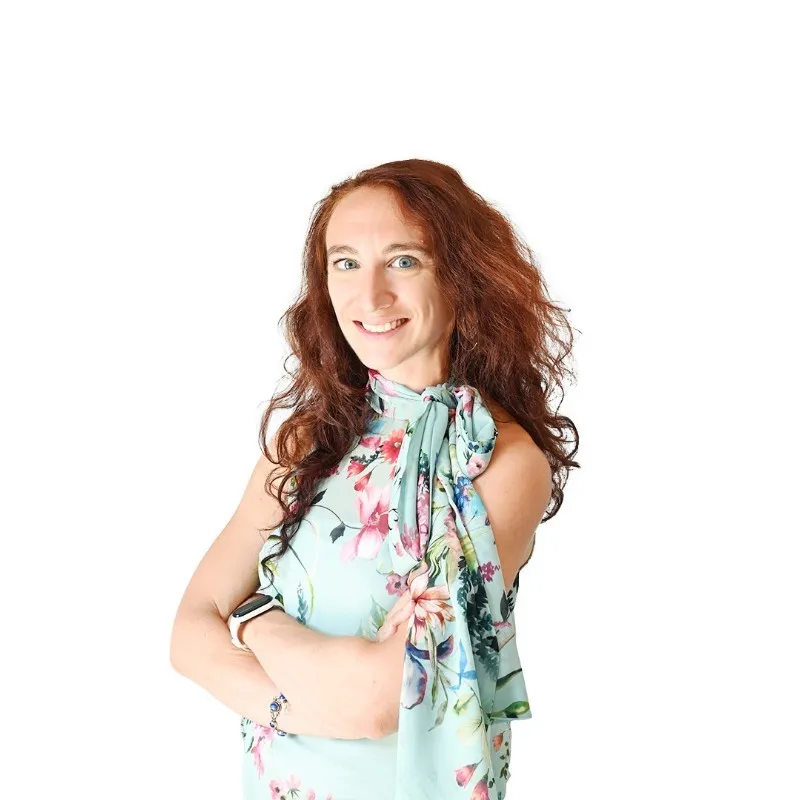
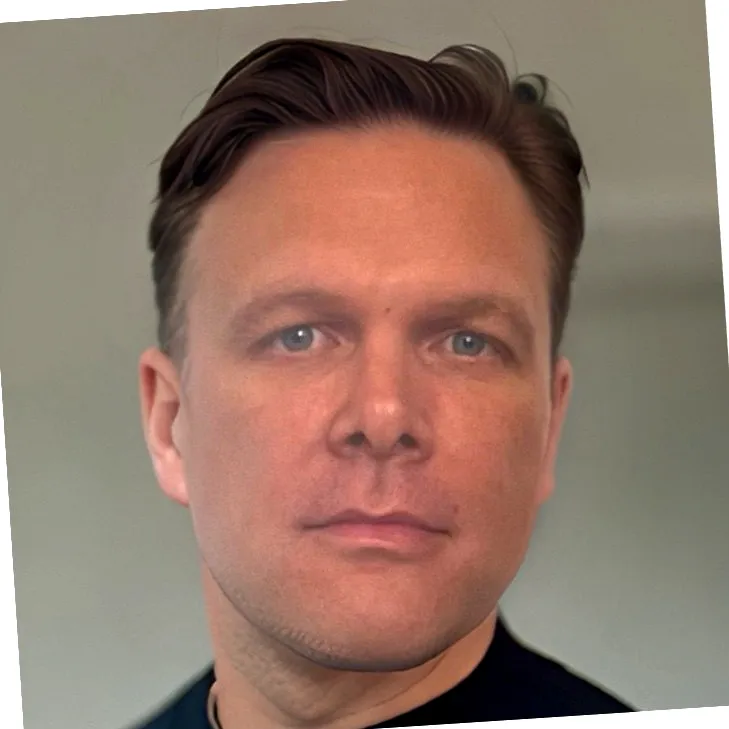
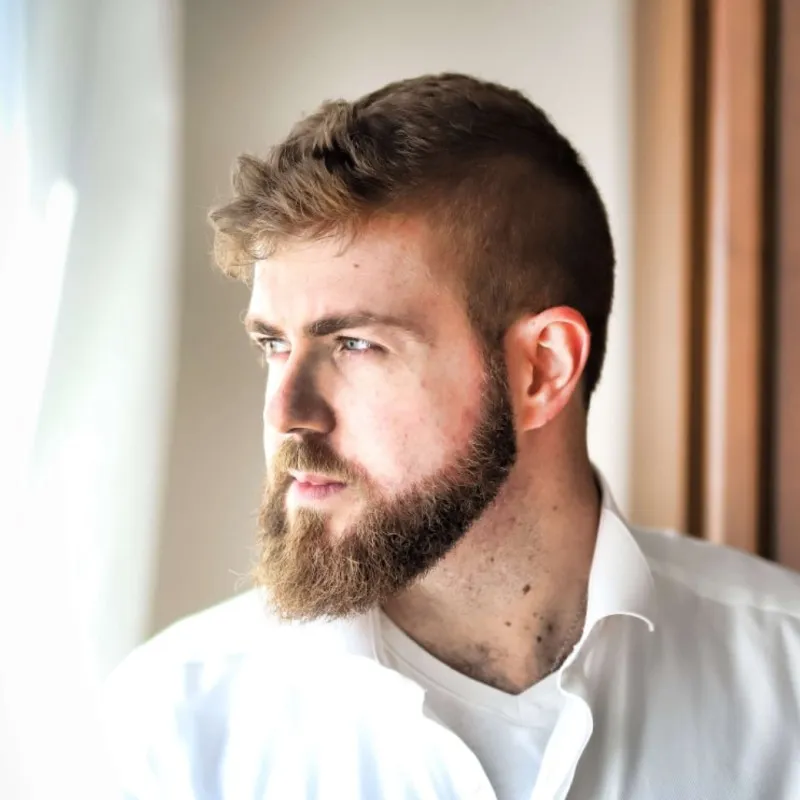
Join Yan, Serena, Anton, Monica, AJ, Natalie, and other devs who are enjoying this book!
Hey, dear serverless friend
- Are you building web APIs with AWS Lambda and you wish there was a way to reduce cold-starts?
- Your app is generating millions of Lambda invocations per day and the cost is starting to add up?
- You know that Lambda is a sustainable platform, but are you wondering if you could do more?
Well, you are in the right place!
We have been building Lambda functions using the Rust programming language for a while now and we have seen impressive results in terms of efficiency, cost, and sustainability. In addition to that, Rust is a fantastic language with a great type system, which makes it easier to write elegant and correct code. Now, we are on a mission to share what we learnt so far!
With this practical e-book you will learn everything there is to know to be able to write, test and ship Lambda Functions written in Rust.
Join us in this journey and let's make the cloud a better place, one Lambda at a time!
What will you get from this book?
Are you looking to build efficient and sustainable AWS serverless solutions that won't break the bank? Wondering how the Rust programming language could help you achieve that? You've come to the right place! Check out this practical guide for all the tips and tricks you need to know to create cost-effective serverless solutions using AWS and Rust.
Hands-on code examples
Learn by doing with practical and realistic code examples that demonstrate how to write efficient and sustainable Lambda functions in Rust.
async fn function_handler(
event: LambdaEvent<EventBridgeEvent<Value>>,
) -> Result<(), Error> {
let start = Instant::now();
let resp = reqwest::get("https://loige.co").await;
let duration = start.elapsed();
let timestamp = event
.payload
.time
.unwrap_or_else(chrono::Utc::now)
.format("%+")
.to_string();
let mut item = HashMap::new();
item.insert(
"Id".to_string(),
AttributeValue::S(format!("{}#{}", "https://loige.co", timestamp)),
);
item.insert("Timestamp".to_string(), AttributeValue::S(timestamp));
let success = match resp {
Ok(resp) => {
let status = resp.status().as_u16();
item.insert("Status".to_string(), AttributeValue::N(status.to_string()));
item.insert(
"Duration".to_string(),
AttributeValue::N(duration.as_millis().to_string()),
);
resp.status().is_success()
}
Err(e) => {
item.insert("Error".to_string(), AttributeValue::S(e.to_string()));
false
}
};
item.insert("Success".to_string(), AttributeValue::Bool(success));
let insert_result = dynamodb_client
.put_item()
.table_name("my_dynamo_table")
.set_item(Some(item))
.send()
.await?;
tracing::info!("Insert result: {:?}", insert_result);
Ok(())
}
Design serverless architectures
It's not just about the Lambda functions! Learn how to design complete serverless architectures using AWS services like API Gateway, EventBridge, DynamoDB, and more using Infrastructure as Code.
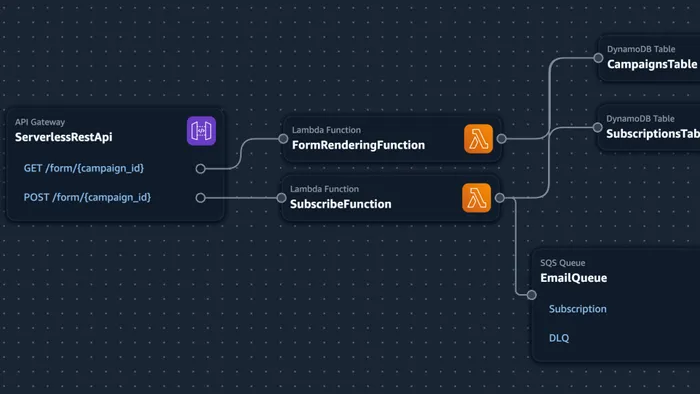
The tools of the trade
Discover the best tools and practices to build, test, and deploy production-grade Lambda functions using Rust.
$ cargo lambda build --release --arm64
What's inside?
This book consists of 15 chapters, each a step forward on your journey to bring Rust and AWS Lambda into production, unlocking gains in performance and cost efficiency. Explore the seamless integration of Rust with Lambda and witness the transformative impact it can have on your projects.
The content is subject to change.
If you get the EARLY-ACCESS bundle, you'll have access to new chapters and updates and you will be able to contribute with your precious feedback.
- Draft
Chapter 1. Rust & Lambda
Why this combo makes a ton of sense compared to other alternatives.
- Draft
Chapter 2. A 'Hello, Serverless' API
Writing our first Rust-powered Lambda Function.
- Draft
Chapter 3. Infrastructure as code with SAM
How to deploy and manage Rust Lambda functions with the Serverless Application Model.
- Draft
Chapter 4. Integrating with external systems
How to make arbitrary HTTP requests or to other AWS services from your Lambda functions.
- Planned
Chapter 5. Code organisation
Best practices on how to organise the code for projects with multiple Lambda functions.
- Planned
Chapter 6. Making the code testable
How to structure the code to be able to write good tests for your Lambda
- Planned
Chapter 7. Handling different kinds of events
How to handle AWS Specific events, custom events and arbitrary JSON in a strongly-typed way.
- Planned
Chapter 8. Different ways of writing Lambda handlers
From simple async functions, to custom structs, to implementing the Tokio Tower service trait.
- Planned
Chapter 9. Configuration management and handling secrets
Best practices to inject configuration and secrets into your Rust Lambda functions.
- Planned
Chapter 10. Observing all the things
How to make our Rust Lambda functions observable using CloudWatch or OpenTelemetry.
- Planned
Chapter 11. Middlewares
How to embrace the middleware pattern to handle cross-cutting concerns in an elegant and reusable way.
- Planned
Chapter 12. Hosting existing HTTP services
How to wrap existing HTTP services in a Lambda function to make them easily available as serverless functions.
- Planned
Chapter 13. Lambda extensions
Writing Lambda extensions using Rust.
- Planned
Chapter 14. Integrating with GitHub Actions
How to configure the integration between AWS and GitHub Actions to test, build and deploy your Lambda Functions in an automated fashion.
- Planned
Appendix A. IaC alternatives
How to use CDK or Terraform as an alternative to SAM.
Who is this book for
This book is designed for cloud developers who are passionate about Serverless and want to build green, efficient, and cost-effective serverless solutions using Rust and AWS Lambda.
This book assumes you have some basic understanding of the Rust programming language. If that's something totally new for you, we'd recommend you to check out the official Rust book (available for FREE) first.
You will also need some basic understanding of AWS and serverless. If you are new to AWS, we recommend you to try some of the FREE Lambda workshops by AWS.
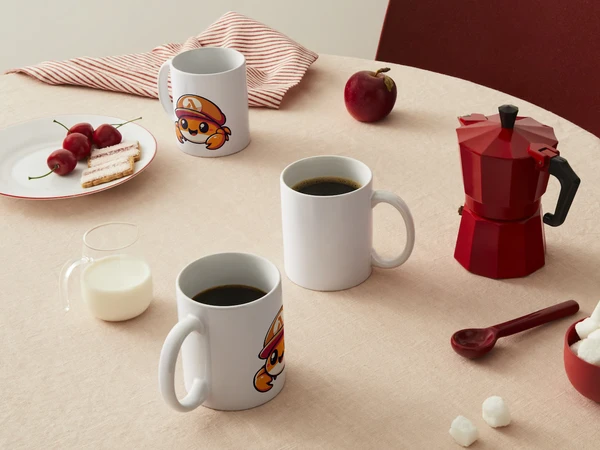
This book is for you if:
- You are a cloud developer with a passion for Serverless
- You have very basic understanding of the Rust programming language
- You have basic knowledge of AWS and Lambda
- You are eager to build greener, efficient, and cost-effective serverless solutions
The early-access bundle
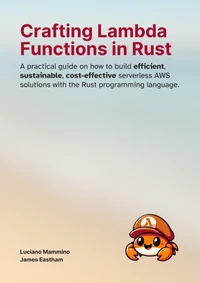
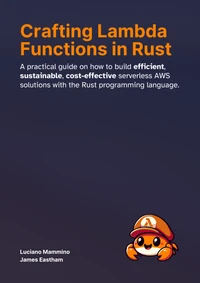
This book is still a work in progress.
So why should you buy it now?
If you get the early-acces bundle, you won't just be supporting the authors but you will also:
- Get the book at the discounted price of
45.9022.95 $! - Get frequent updates in ePub and PDF in both light and dark mode!
- Get access to our exclusive Discord server.
- Have a chance to review, provide early feedback and get your name in the credits.
- Get the unconditioned love of the authors! ♥︎
What people are saying
Don't just listen to us. Listen to them.
Hopefully we will have some lovely reviews here... soon! 😇.
Do you want to help? Leave us a review on Gumroad!
FAQs
Yes, this book is designed to be accessible to readers with very basic knowledge of Rust and AWS. The early chapters provide a gentle introduction to Lambda functions and serverless computing in a simple and accessible way, catering to the needs of readers from different backgrounds.
No. While a basic understanding of Rust syntax is helpful, this book is beginner-friendly. If you are new to Rust, reading the official Rust book first might be beneficial. The book explains advanced Rust concepts as they are used in the context of AWS Lambda.
No. This book assumes only a basic familiarity with AWS. You should have an AWS account and have the AWS CLI installed and configured on your development machine. The book covers all necessary AWS serverless concepts and services, including DynamoDB, API Gateway, EventBridge, CloudWatch, SAM, and more in a way that is suitable to AWS beginners. Even if you have never used these services and tools, you should be able to follow along and understand the concepts as you progress through the book.
No. This book balances theory and practice. You’ll build real applications, discuss technical trade-offs, and progressively enhance your examples into production-ready applications. By the end, you’ll have the knowledge needed to create production-ready serverless applications on AWS using Rust.
Yes, all the code examples, including solutions to the exercises, are freely available on GitHub.
Yes. Each chapter ends with challenges and exercises to reinforce the concepts learned. If you get stuck, solutions are available along with the book’s code examples.